Allowing Users to send email with their own SMTP settings in Laravel
Published on by Andrés Santibáñez
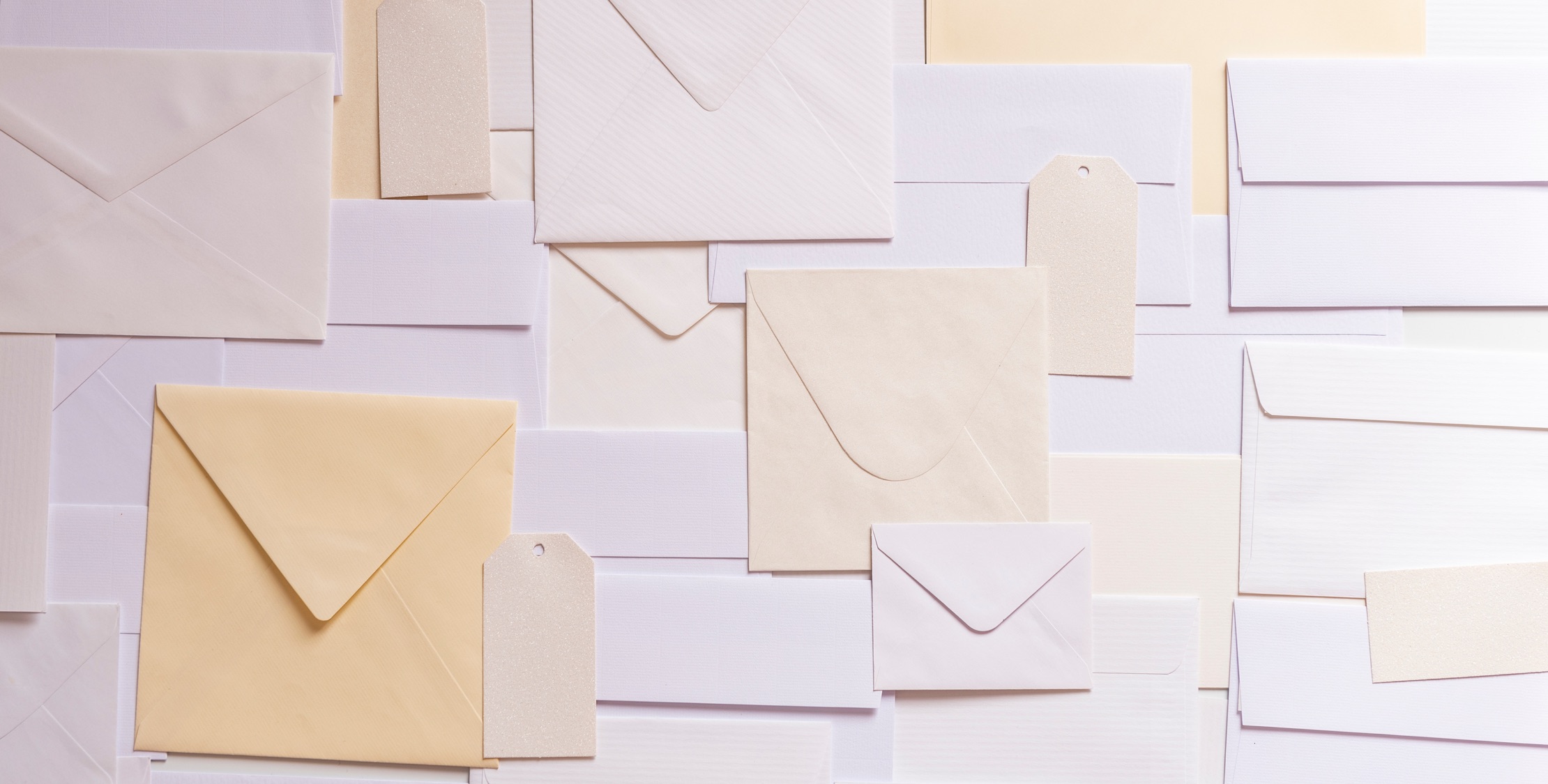
In a recent project, I needed a way to allow users to send emails with their SMTP credentials. The goal with this was to give all the outgoing emails a more personal touch and look like it came directly from an organization versus a generic one for the web app.
I started looking for options and packages that would deliver this kind of functionality since I’d only sent emails in my projects configuring the provided mail environment variables available in any Laravel project.
MAIL_DRIVER=MAIL_HOST=MAIL_PORT=MAIL_USERNAME=MAIL_PASSWORD=MAIL_ENCRYPTION=
Setting up these environment variables with valid SMTP credentials would give you the ability to send emails in no time in your project. However, in my case, I needed to switch these credentials at runtime depending on the logged in user. The solution wasn’t a good fit because the framework configures the Mail
facade once with the provided environment variables and each call to Mail::send()
will use these credentials. The Mail
facade configuration can be seen in the framework’s MailServiceProvider
class, specifically in the registerIlluminateMailer() method where it configures a singleton instance of the Mailer
class. This Mailer
instance is used each time we use the Mail
facade.
Laravel’s Mail facade is a joy to work with. Configuring recipients and email content is done with a simple and intuitive API. Since I wanted to achieve the same expressiveness when sending emails with runtime configured credentials, I looked into how the Mailer
instance was configured by the framework and how I could get an instance of that class but with other SMTP credentials.
Digging deeper into the Mailer
class, I found out that a new instance can be built with a Swift_Mailer
object. In turn, the Swift_Mailer
class is built with a Swift_SmtpTransport
object which houses the SMTP credentials that will be used to send emails. Both Swift_Mailer
and Swift_SmtpTransport
are part of the SwiftMailer package Laravel uses under the hood for sending emails.
With this chain of dependencies figured out, I needed a way to build these objects to be able to specify the email credentials I wanted to use. First, we set up a new Swift_SmtpTransport
object with our credentials.
$transport = new Swift_SmtpTransport('SMTP-HOST-HERE', SMTP-PORT-HERE);$transport->setUsername('SMTP-USERNAME-HERE');$transport->setPassword('SMTP-PASSWORD-HERE'); // extra configurations here if needed
Then, we use the Swift_SmtpTransport
to build our Swift_Mailer
object.
$swift_mailer = new Swift_Mailer($transport);
With these two objects in place, we now need a Mailer
object with our new configuration. As seen in the MailServiceProvider
class, the Mailer
object is built using three parameters: A view factory, the Swift_Mailer
and an optional Event Dispatcher. We can get the extra parameters thanks to Laravel’s Service Container via the app()->get()
helper.
$view = app()->get('view');$events = app()->get('events');$mailer = new Mailer($view, $swift_mailer, $events);
We also set up the replyTo and from properties, so each email sent with the Mailer
has these signatures.
$mailer->alwaysFrom('from-email', 'from-name');$mailer->alwaysReplyTo('from-email', 'from-name');
With the new Mailer
object, we can now send emails with our runtime configured credentials.
$mailer->to('recipient')->send(new SomeMailable());
Simplifying our custom Mailer setup
Now that we have our custom Mailer
setup, we can put this code in a Service class or in a function that will return us the Mailer
object ready to be used. However, a more elegant way to get our custom Mailer
would be using Laravel’s Service Container to build our object every time we need it. In our project’s AppServiceProvider
class, we can register a new binding with any key we would like to use to get our custom Mailer
. In my case, I used user.mailer
as a key.
// AppServiceProvider.class public function register(){ $this->app->bind('user.mailer', function ($app, $parameters) { $smtp_host = array_get($parameters, 'smtp_host'); $smtp_port = array_get($parameters, 'smtp_port'); $smtp_username = array_get($parameters, 'smtp_username'); $smtp_password = array_get($parameters, 'smtp_password'); $smtp_encryption = array_get($parameters, 'smtp_encryption'); $from_email = array_get($parameters, 'from_email'); $from_name = array_get($parameters, 'from_name'); $from_email = $parameters['from_email']; $from_name = $parameters['from_name']; $transport = new Swift_SmtpTransport($smtp_host, $smtp_port); $transport->setUsername($smtp_username); $transport->setPassword($smtp_password); $transport->setEncryption($smtp_encryption); $swift_mailer = new Swift_Mailer($transport); $mailer = new Mailer($app->get('view'), $swift_mailer, $app->get('events')); $mailer->alwaysFrom($from_email, $from_name); $mailer->alwaysReplyTo($from_email, $from_name); return $mailer; });}
With the binding in place, every time we need our customer Mailer
we can use Service Container to build an instance for us passing the necessary configuration.
$configuration = [ 'smtp_host' => 'SMTP-HOST-HERE', 'smtp_port' => 'SMTP-PORT-HERE', 'smtp_username' => 'SMTP-USERNAME-HERE', 'smtp_password' => 'SMTP-PASSWORD-HERE', 'smtp_encryption' => 'SMTP-ENCRYPTION-HERE', 'from_email' => 'FROM-EMAIL-HERE', 'from_name' => 'FROM-NAME-HERE',]; $mailer = app()->makeWith('user.mailer', $configuration); // Use $mailer here...
Caveats
In Laravel, Mailables
can be queued to be sent later by our project’s configured queue system. Queued Mailables
are always dispatched with the Mail
facade meaning that if we queued an email with our custom Mailer
, it would be sent with the project’s mail credentials instead of our custom ones. To prevent this from happening, we can set up each Mailable that will use our custom credentials to not be queueable by default. To do this, we should remove the ShouldQueue
implementation from our Mailable
classes.
Change
class SomeMailable extends Mailable implements ShouldQueue{ // Mailable code here}
to
class SomeMailable extends Mailable{ // Mailable code here}
Next, we will create a Job class that will be queuable and internally uses our custom Mailer
. We will call this class UserMailerJob
which will receive our custom configuration, the email recipients and the Mailable
object to be sent.
class UserMailerJob implements ShouldQueue{ use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public $configuration; public $to; public $mailable; /** * Create a new job instance. * * @param array $configuration * @param string $to * @param Mailable $mailable */ public function __construct(array $configuration, string $to, Mailable $mailable) { $this->configuration = $configuration; $this->to = $to; $this->mailable = $mailable; } /** * Execute the job. * * @return void */ public function handle() { $mailer = app()->makeWith('user.mailer', $this->configuration); $mailer->to($this->to)->send($this->mailable); }}
With this job in place, we can dispatch our UserMailerJob
job to send Mailables
with our project’s queue system.
$configuration = [ 'smtp_host' => 'SMTP-HOST-HERE', 'smtp_port' => 'SMTP-PORT-HERE', 'smtp_username' => 'SMTP-USERNAME-HERE', 'smtp_password' => 'SMTP-PASSWORD-HERE', 'smtp_encryption' => 'SMTP-ENCRYPTION-HERE', 'from_email' => 'FROM-EMAIL-HERE', 'from_name' => 'FROM-NAME-HERE',]; UserMailerJob::dispatch($configuration, 'recipient', new SomeMailable());
Conclusion
With this setup, we can send emails with custom credentials configured at runtime. What’s nice about this implementation is that we haven’t reached out for any packages that solve this particular problem and that we have used the same objects and orchestration Laravel uses under the hood to send emails.
This is one of the many possible implementations one can do to solve this type of requirement.
Web and Mobile dev. Father of two. Married to the best wife ever. Enjoying every little moment life gives us.