Version 5.4 of Laravel is now officially released! This release has many new features, and improvements and here is quick video going over some of the highlights:
Laravel Dusk
Laravel Dusk is an end-to-end browser testing tool for JavaScript enabled applications. It aims to provide the right way to do page interaction tests, so you can use Dusk for things like click buttons/links, forms, as well as drag and drop!
Dusk utilizes the ChromeDriver and the Facebook Php-webdriver for tests. It can work with any Selenium browser, but comes with ChromeDriver by default which will save you from installing a JDK or Selenium.
Dusk is very easy to use without setting up Selenium and starting the server every time.
Laravel Mix
Laravel Mix is the next generation of Elixir. It is built with webpack, instead of Gulp. It was renamed because of the significant changes.
Unless you customized your Elixir setup, moving to Mix shouldn’t be a problem and Laracasts has a video covering this updated tool.
Blade Components and Slots
Components and Slots are designed to give you even more flexibility in your Blade templates. As an example, imagine you have an include template that is used for showing an alert:
// alert.blade.php<div class="alert"> {{ $slot }}</div>
Then, in your template file you can include it like this:
@component('inc.alert') This is the alert message here.@endcomponent
Markdown Emails
Laravel 5.3 introduced two new features around email, Mailables and Notifications which allow you to send the same message through email, SMS, and other channels.
Building on top of these improvements, Laravel 5.4 includes a brand new Markdown system for creating email templates.
Under the hood, this feature implements the Parsedown parser with its companion, Markdown Extra so you can use tables.
@component('mail:message') # Thank You Thank you for purchasing from our store. @component('mail::button', ['url' => $actionUrl, 'color' => $color]){{ $actionText }}@endcomponent @endcomponent
Automatic Facades
You can now use any class as a Facade on the fly. Here is an example:
namespace App; class Zonda{ public function zurf() { return ‘Zurfing’; }}
Then, in your routes or controller:
use Facades\ { App\Zonda}; Route::get('/', function () { return Zonda::zurf();});
Route Improvements
Another new feature is the ability to use fluent syntax to define a named route or a middleware:
Route::name('profile')->get('user/{id}/profile', function ($id) { // some closure action...}); Route::name('users.index')->middleware('auth')->get('users', function () { // some closure action...}); Route::middleware('auth')->prefix('api')->group(function () { // register some routes...}); Route::middleware('auth')->resource('photo', 'PhotoController');
The route caching layer also received improvements which will allow route matching on very large applications to see a significant enhancement.
Higher Order Messaging for Collections
The best way of showcasing this new feature is through code samples. Pretend you have a collection, and you want to perform an operation on each of the items:
$invoices->each(function($invoice) { $invoice->pay();});
Can now become:
$invoices->each->pay();
More New Features
Some other changes and improvements include the following:
- New
retry
helper - New
array_wrap
helper - Added a default 503 error page
- Switched to the
::class
notation through the core. - Added names to password reset routes
- Support for PhpRedis
- Added IPv4 and IPv6 validators
-
date_format
validation is now more precise
Upgrading to Laravel 5.4
The official docs include a full upgrade guide, and there are some of the changes you should be aware of.
Laravel Tinker is now a stand-alone package, and installation is simple. Require the package and include the service provider:
composer require laravel/tinker
When that finishes, add the service provider to your config/app.php file:
Laravel\Tinker\TinkerServiceProvider
Your existing tests that utilize browser kit will either need to be migrated to Laravel Dusk or include the older package:
composer require laravel/browser-kit-testing --dev
To get the latest version modify your composer.json
file and change the laravel/framework
dependency to 5.4.*
.
Learning More About Laravel 5.4
Laracasts has a complete series available on all these new features, and the official docs has the upgrade guide as well as the release notes.
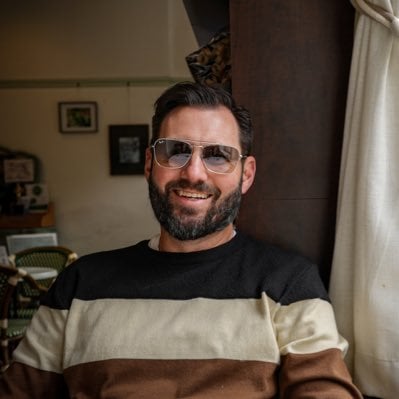
Eric is the creator of Laravel News and has been covering Laravel since 2012.