Laravel 9.42 comes with new singleton route resources, conditional report helpers, and more
Published on by Paul Redmond
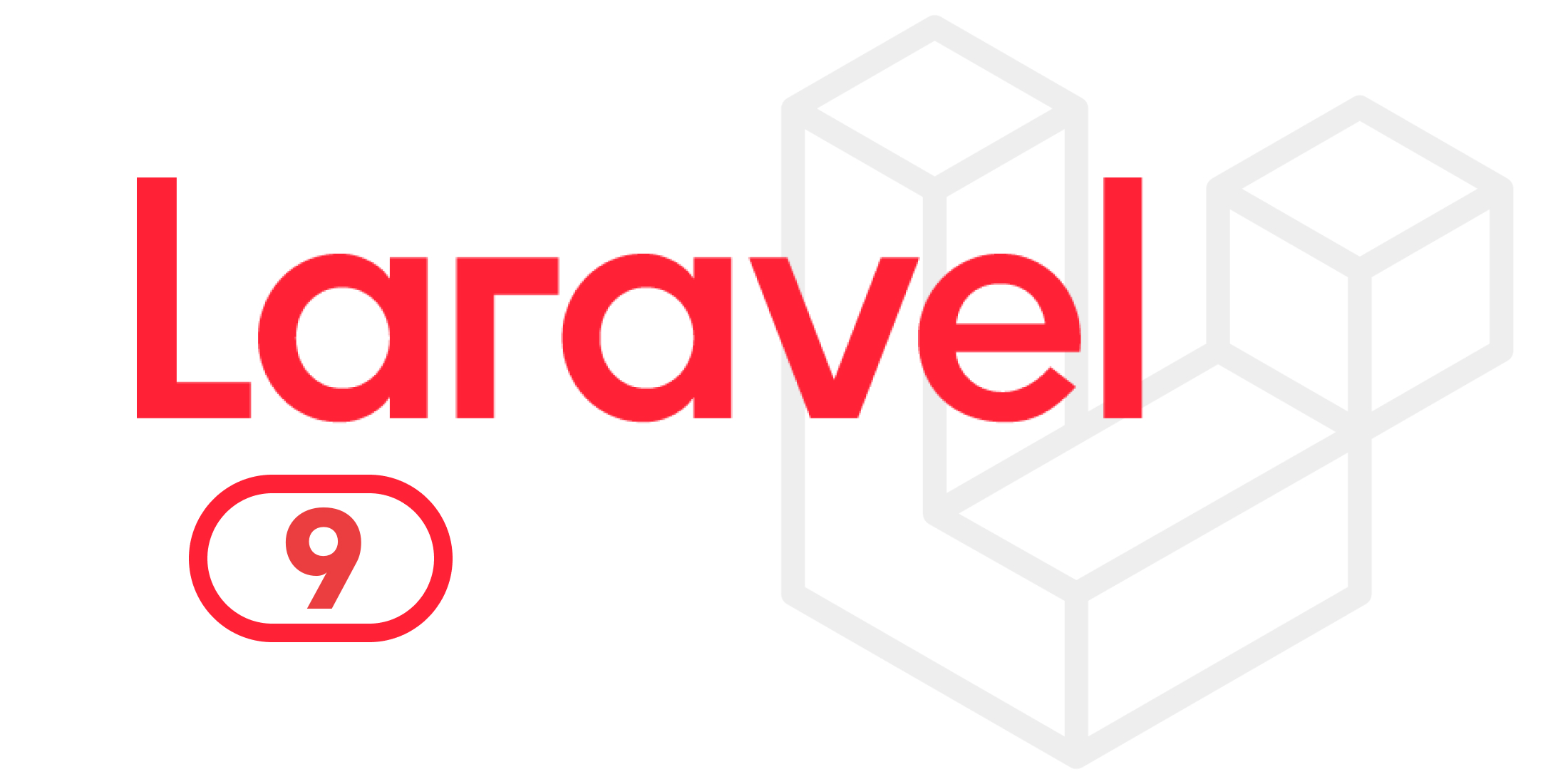
The Laravel team released 9.42 this week with singleton route resources, conditional report helpers, and more:
Thanks to @jessarchercodes, today's Laravel release allows you to quickly define routes for "singleton" resources.
— Taylor Otwell 🪐 (@taylorotwell) November 29, 2022
These are resources like a user "profile" that have one and only one instance in your application.
📕 Read more in the docs: https://t.co/IyAvu0eYWz pic.twitter.com/WSD4vgSiBY
Define routes for singleton resources
Jess Archer contributed a Route::singleton()
method to register routes for singleton resources. These are resources that are either "one of one" or "zero or one" resources. For example, a profile
endpoint:
Route::singleton('profile', ProfileController::class);/*Defines:GET /profileGET /profile/editPUT/PATCH /profileDELETE /profile*/ // Nested resourceRoute::singleton('videos.thumbnail', VideoThumbnailController::class); /*Defines:GET /videos/{video}/thumbnailGET /videos/{video}/thumbnail/editPUT/PATCH /videos/{video}/thumbnailDELETE /videos/{video}/thumbnail*/ // Only and exceptRoute::singleton('profile', ProfileController::class) ->except('destroy'); Route::singleton('profile', ProfileController::class) ->only(['show', 'update']);
The make:controller
command was also updated to include --singleton
and --creatable
flags to generate singleton controllers.
Check out the Singleton Resource Controllers docs for more examples and details. Excellent work, Jess!
Added a rest option for queue:listen
@PHPGuus contributed a --rest=
option to the queue:listen
command that will sleep for the number of seconds passed to rest:
php artisan queue:listen --rest=5
Determine if a string is a ULID
Michael Nabil contributed an isUlid()
method to the Stringable
class to check if a string is a valid Universally Unique Lexicographically Sortable Identifier (ULID) string. You can use it as follows:
use Illuminate\Support\Str; Str::isUlid('01GJSNW9MAF792C0XYY8RX6QFT'); // trueStr::of('01GJSNW9MAF792C0XYY8RX6QFT')->isUlid(); // true
Conditional report helpers
Michael Nabil contributed two new conditional report()
helpers. These might be useful to shorten checks where you might report when a condition is true
or false
:
// Normal report usageif(! Auth::user()->isAdmin()){ report('Is route need permission user');} // Shorten/avoid `if` with:report_if( Auth::user()->isAdmin(), 'Is route need permission admin'); report_unless( Auth::user()->isAdmin(), 'Is route need permission user');
These helpers are optional—you are free to use them or not :)
Custom mutex name of scheduled events
Andrej Mihaliak contributed a callback to resolve a custom mutex name of scheduled events:
$schedule->command('do-something', ['--uuid' => 'b4d5f926-e1b5-4285-b068-cf1d623a0635']) ->description('Do something important.') ->everyFiveMinutes() ->onOneServer() ->withoutOverlapping() ->createMutexNameUsing(fn (Event $event) => 'schedule-task-'.sha1($event->description));
Check out Pull Request #45126 for complete details.
Release Notes
You can see the complete list of new features and updates below and the diff between 9.41.0 and 9.42.0 on GitHub. The following release notes are directly from the changelog:
v9.42.0
Added
- Added --rest option to queue:listen (00a12e2, 82fde9e)
- Added
Illuminate/Support/Stringable::isUlid()
(#45100) - Add news report_if and report_unless helpers functions (#45093)
- Add callback to resolve custom mutex name of schedule events (#45126)
- Add WorkOptions to WorkerStopping Event (#45120)
- Added
singleton
andcreatable
options toIlluminate/Routing/Console/ControllerMakeCommand
(#44872)
Fixed
- Fix pure enums validation (#45121)
- Prevent test issues with relations with the $touches property (#45118)
- Fix factory breaking when trying to determine whether a relation is empty (#45135)