Quickly test the performance of your Laravel app with the Benchmarking helper
Published on by Paul Redmond
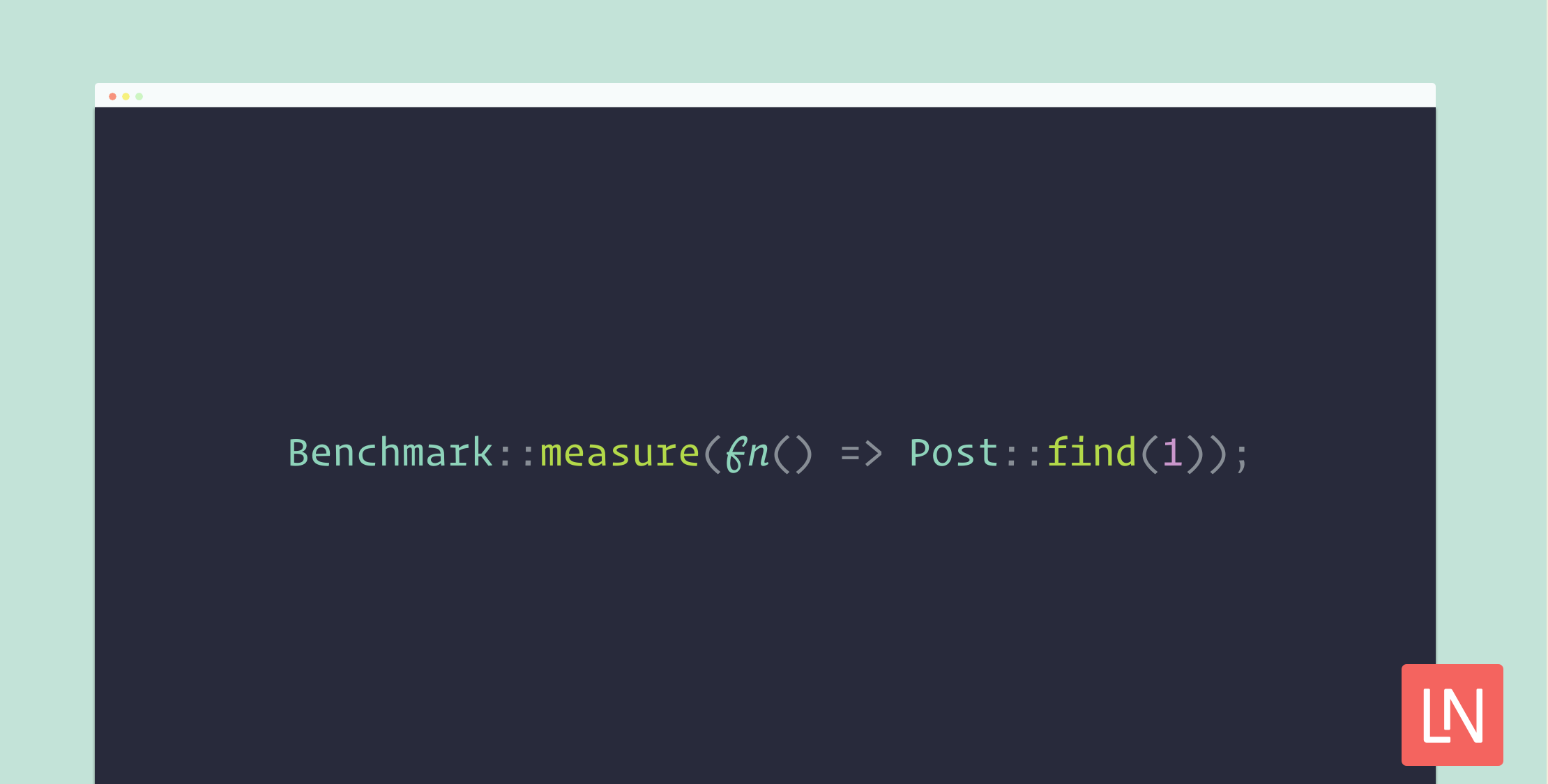
With the release of Laravel 9.32 yesterday, a benchmarking helper was introduced, which is useful to quickly test the performance of certain parts of your application.
You thought that yesterday was only about the new "dd" source stuff? There is more! Beginning with yesterday's @laravelphp release, you may use the new "Benchmark" class to quickly test the performance of certain parts of your application. ⏱
— nuno maduro 🤌🏻 (@enunomaduro) September 29, 2022
📚 https://t.co/7G7B5mJuVx. pic.twitter.com/JeYVk9m5Tm
It works by passing a Closure
that runs some code you want to benchmark and returns the time it took in ms
:
use Illuminate\Support\Benchmark; Benchmark::measure(fn() => Post::find(1));// Returns time in ms.// i.e., 0.1ms
Additionally, you can pass an array of Closure
s and optionally configure how many iterations the closures should run:
// Run each callback three timesBenchmark::measure([ fn() => Post::find(1), fn() => Post::find(5),], 3); // [0.02, 0.03] // Use keysBenchmark::measure([ 'Post 1' => fn() => Post::find(1), 'Post 5' => fn() => Post::find(5),], 3);// ['Post 1' => 0.02, 'Post 5' => 0.03]
The Benchmark class has a dd()
method which runs the above measurements wrapped with a dd()
call, which will output the results to the console or browser and exit.
Benchmark::dd([ 'Post 1' => fn() => Post::find(1), 'Post 5' => fn() => Post::find(5),]);
Couple this update with the dd()
file/line output, and you have some useful new debugging tools!
To learn more, check out the benchmarking section now available within the helpers documentation.