New in Laravel 5.3.17 is an improvement to Model Factories that you allows to define different “states” for a factory.
For example pretend you have a simple faker for creating a User:
$factory->define(App\User::class, function (Faker\Generator $faker) { return [ 'name' => $faker->name, 'email' => $faker->safeEmail, ];});
Now you want to have the ability to define an administrator:
$factory->state(\App\User::class, 'admin', function (\Faker\Generator $faker) { return [ 'is_admin' => 1, ];});
Then a moderator flag:
$factory->state(\App\User::class, 'moderator', function (\Faker\Generator $faker) { return [ 'is_moderator' => 1, ];});
With these three set you can now call them like this:
// Create 5 usersfactory(\App\User::class, 5)->create(); // Create 5 Adminsfactory(\App\User::class, 5)->states('admin')->create(); // Create 5 Moderatorsfactory(\App\User::class, 5)->states('moderator')->create(); // Create 5 Admins that are also moderatorsfactory(\App\User::class, 5)->states('admin', 'moderator')->create();
Changes
Also, included with this release is a change to the collection only method:
-
Collection::only()
now returns all items if$keys
isnull
(#15695)
Bug Fixes:
- Added workaround for Memcached 3 on PHP7 when using
many()
(#15739) - Fixed bug in
Validator::hydrateFiles()
when removing the files array (#15663) - Fixed model factory bug when
$amount
is zero (#15764, #15779) - Prevent multiple notifications getting sent out when using the
Notification
facade (#15789)
The full Laravel changelog is available in the GitHub repo.
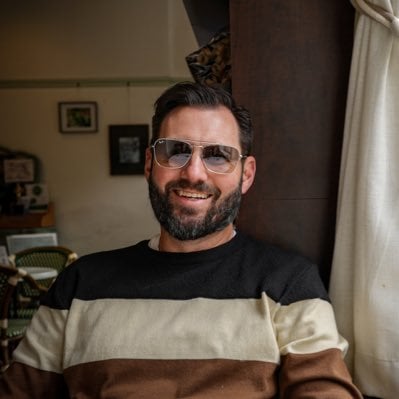
Eric is the creator of Laravel News and has been covering Laravel since 2012.