Need to remove keys from an array or object? Use the data forget helper
Published on by Eric L. Barnes
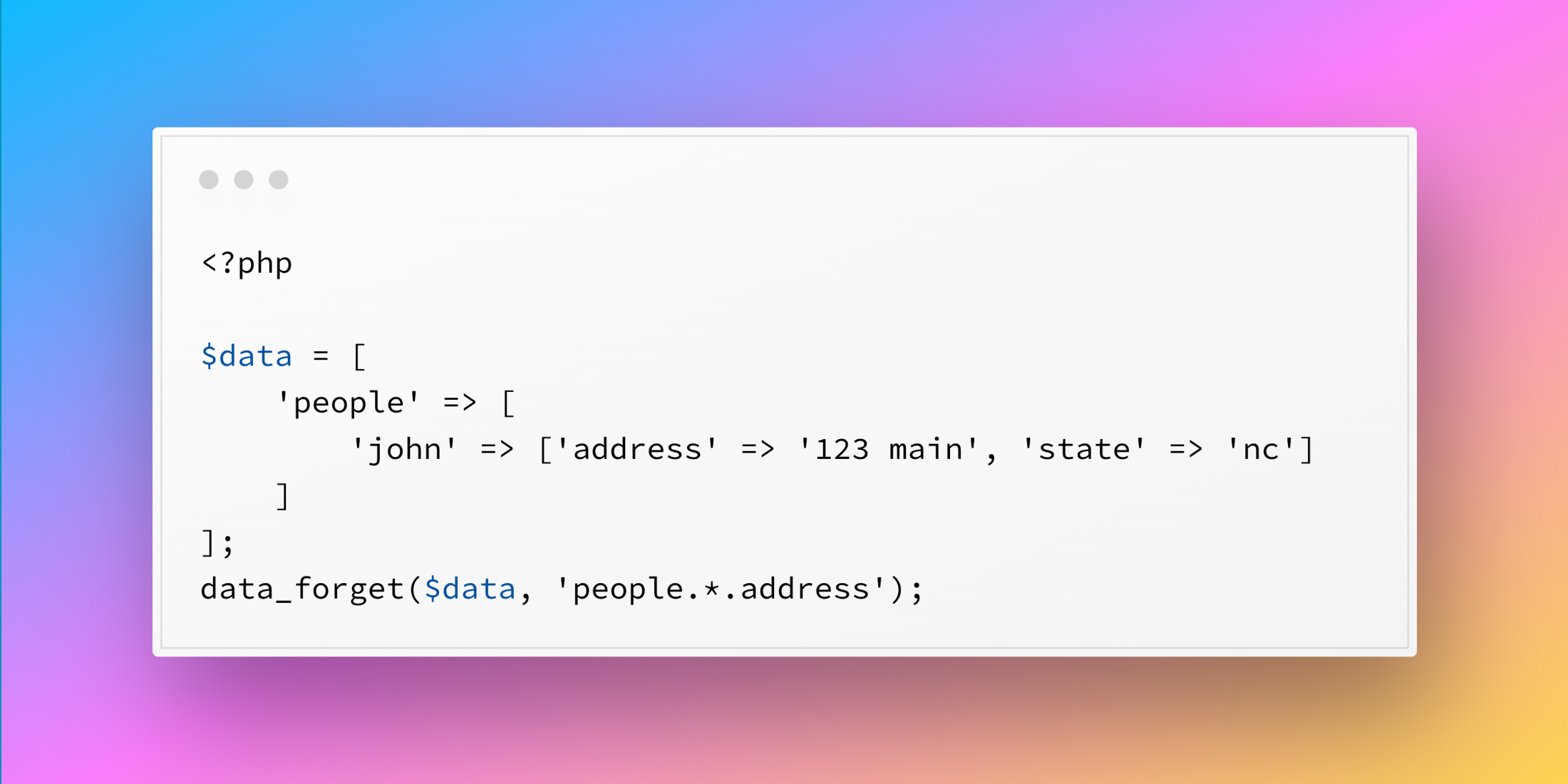
Laravel includes a new utility function called data_forget
that allows you to remove keys from an array or object using "dot" notation, and is included since Laravel v10.15.
Let's take a look at how the data_forget
helper works:
$data = [ 'people' => [ 'john' => ['address' => '123 main', 'state' => 'nc'], 'michael' => ['address' => '34 east 5th', 'state' => 'ny'] ]]; data_forget($data, 'people.*.address');
The data_forget
function removes the 'address' attribute from both the "john" and "jane" sub-array. The result will then be:
[ 'people' => [ 'john' => ['state' => 'nc'], 'michael' => ['state' => 'ny'] ]];
One of the neat features of the data_forget
helper is its flexibility. It supports wildcard characters and complete 'dot' notation.
For instance, in the example above, the wildcard character (*) is used in the key string, specifying any sub-key under 'name'. Alternatively, if you need to remove a key from a specific sub-array, you can do so using the complete 'dot' notation, like so:
data_forget($data, 'people.john.address');
To learn more about the data_forget
helper function, you can refer to the PR on Github.
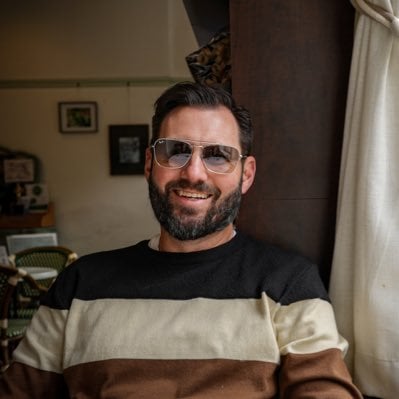
Eric is the creator of Laravel News and has been covering Laravel since 2012.