Laravel Prompts Adds a Multi-line Textarea Input, Laravel 11.3 Released
Last updated on by Paul Redmond
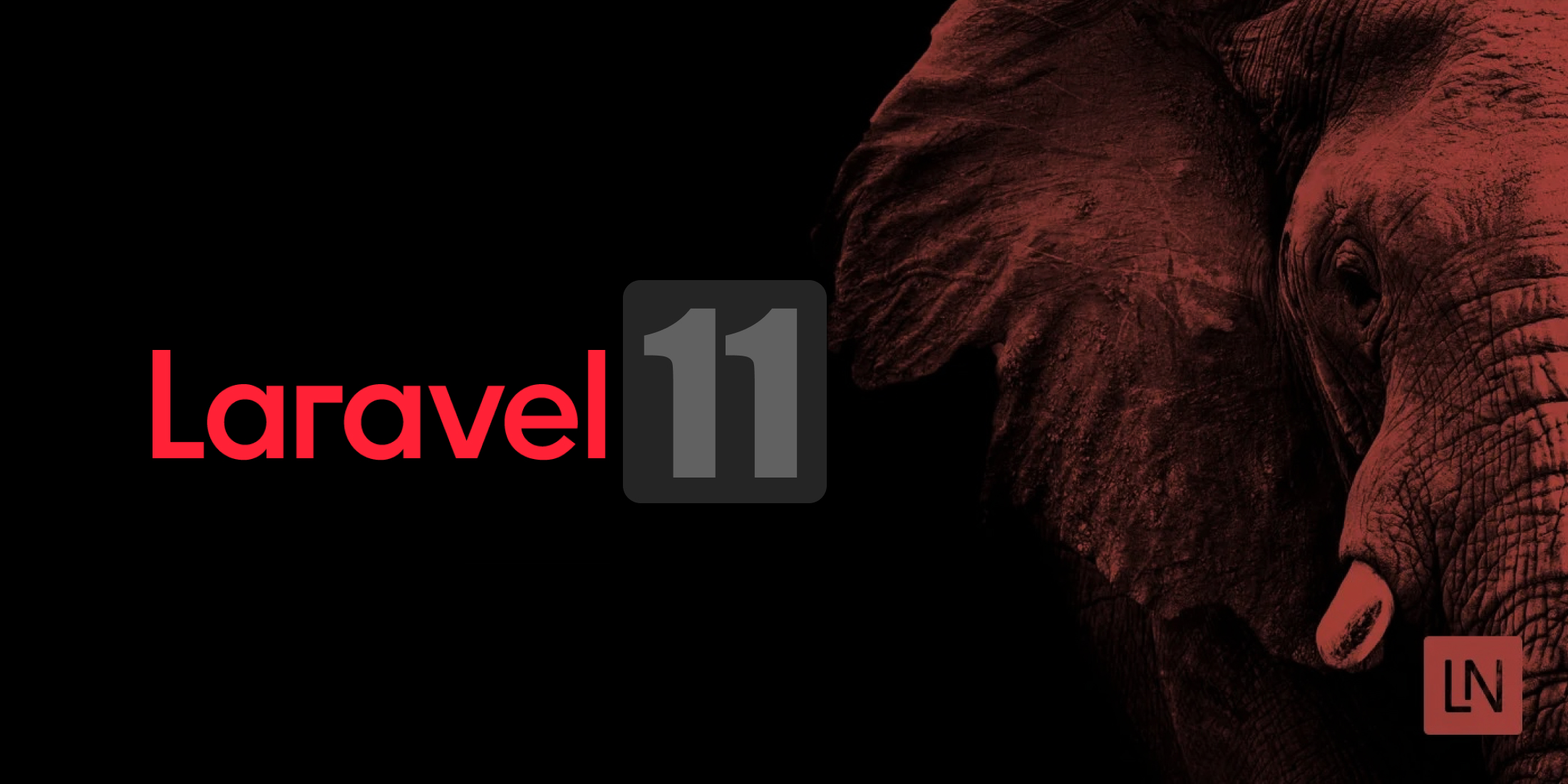
This week, the Laravel team released v11.3, which includes multi-line text in Laravel Prompts, a Session:hasAny() method, a Context::pull() method, and more.
Multi-line Text Prompts
Joe Tannenbaum contributed a textarea function to Laravel prompts that accepts multi-line text from a user:
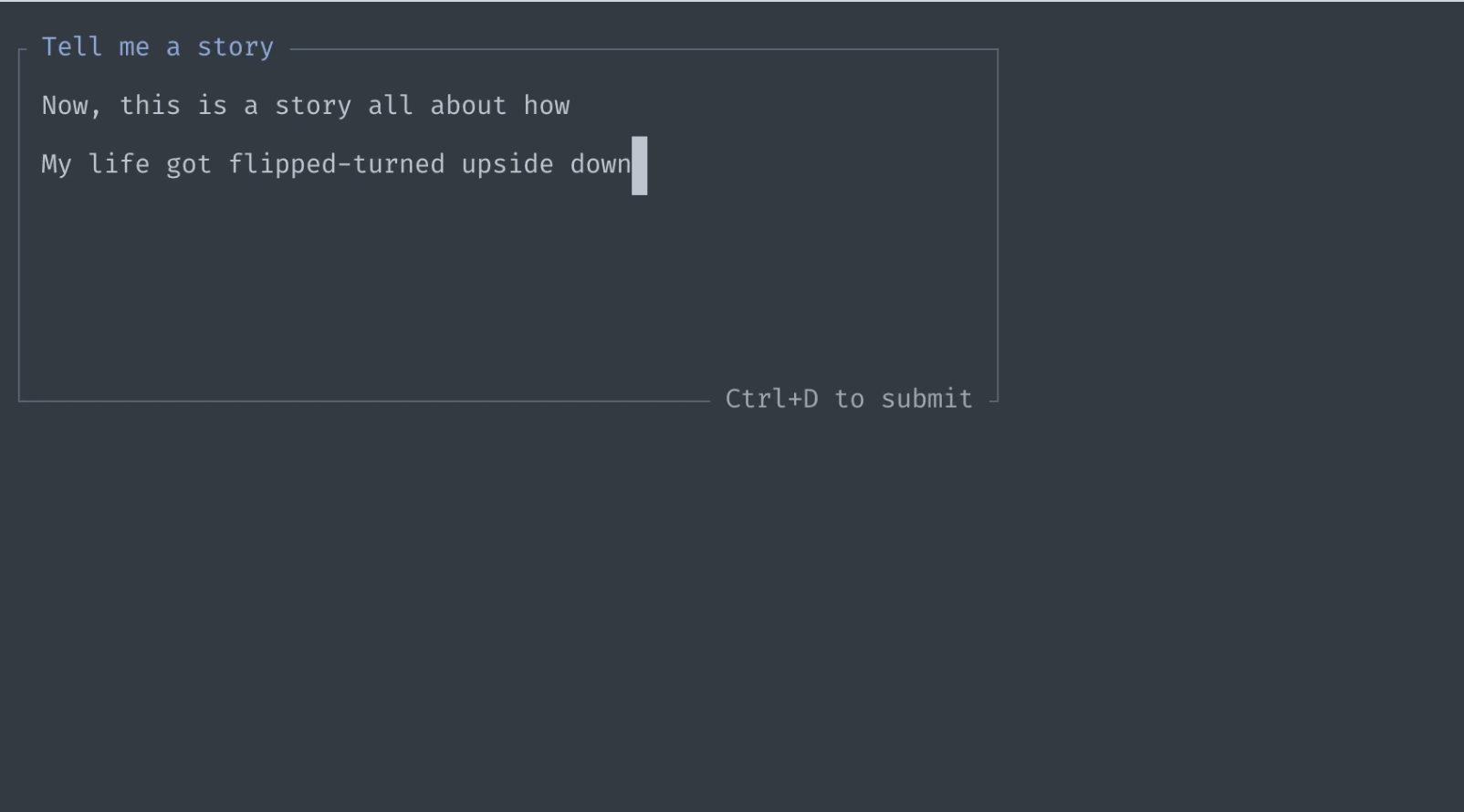
The textarea()
function includes an optional validation argument as well as a required argument to make sure the textarea is filled out:
use function Laravel\Prompts\textarea; $story = textarea( label: 'Tell me a story.', placeholder: 'This is a story about...', required: true, hint: 'This will be displayed on your profile.'); // Validation$story = textarea( label: 'Tell me a story.', validate: fn (string $value) => match (true) { strlen($value) < 250 => 'The story must be at least 250 characters.', strlen($value) > 10000 => 'The story must not exceed 10,000 characters.', default => null });
See the textarea()
function documentation for usage details and Pull Request #88 in the laravel/prompts repository for the implementation.
New Session hasAny() Method
Mahmoud Mohamed Ramadan contributed a hasAny()
method to sessions, which is a nice improvement when checking to see if any values are in the session:
// Beforeif (session()->has('first_name') || session()->has('last_name')) { // do something...} // Using the new hasAny() methodif (session()->hasAny(['first_name', 'last_name'])) { // do something...}
Context Pull Method
@renegeuze contributed a pull()
and pullHidden()
method to the Context service, which pulls the contextual data and immediately removes it from context.
$foo = Context::pull('foo');$bar = Context::pullHidden('foo');
An example use-case for this feature might be capturing context for database logging and pulling it because the additional context is no longer needed.
Release notes
You can see the complete list of new features and updates below and the diff between 11.2.0 and 11.3.0 on GitHub. The following release notes are directly from the changelog:
v11.3.0
- [10.x] Prevent Redis connection error report flood on queue worker by @kasus in https://github.com/laravel/framework/pull/50812
- [11.x] Optimize SetCacheHeaders to ensure error responses aren't cached by @MinaWilliam in https://github.com/laravel/framework/pull/50903
- [11.x] Add session
hasAny
method by @mahmoudmohamedramadan in https://github.com/laravel/framework/pull/50897 - [11.x] Add option to report throttled exception in ThrottlesExceptions middleware by @JaZo in https://github.com/laravel/framework/pull/50896
- [11.x] Add DeleteWhenMissingModels attribute by @Neol3108 in https://github.com/laravel/framework/pull/50890
- [11.x] Allow customizing TrimStrings::$except by @grohiro in https://github.com/laravel/framework/pull/50901
- [11.x] Add pull methods to Context by @renegeuze in https://github.com/laravel/framework/pull/50904
- [11.x] Remove redundant code from MariaDbGrammar by @hafezdivandari in https://github.com/laravel/framework/pull/50907
- [11.x] Explicit nullable parameter declarations to fix PHP 8.4 deprecation by @Jubeki in https://github.com/laravel/framework/pull/50922
- [11.x] Add setters to cache stores by @stancl in https://github.com/laravel/framework/pull/50912
- [10.x] Laravel 10x optional withSize for hasTable by @apspan in https://github.com/laravel/framework/pull/50888
- [11.x] Fix prompting for missing array arguments on artisan command by @macocci7 in https://github.com/laravel/framework/pull/50850
- [11.x] Add strict-mode safe hasAttribute method to Eloquent by @mateusjatenee in https://github.com/laravel/framework/pull/50909
- [11.x] add function to get faked events by @browner12 in https://github.com/laravel/framework/pull/50905
- [11.x]
retry
func - catch "Throwable" instead of Exception by @sethsandaru in https://github.com/laravel/framework/pull/50944 - chore: remove repetitive words by @findseat in https://github.com/laravel/framework/pull/50943
- [10.x] Add
serializeAndRestore()
toNotificationFake
by @dbpolito in https://github.com/laravel/framework/pull/50935 - [11.x] Prevent crash when handling ConnectionException in HttpClient retry logic by @shinsenter in https://github.com/laravel/framework/pull/50955
- [11.x] Remove unknown parameters by @naopusyu in https://github.com/laravel/framework/pull/50965
- [11.x] Fixed typo in PHPDoc
[@param](https://github.com/param)
by @naopusyu in https://github.com/laravel/framework/pull/50967 - [11.x] Fix dockblock by @michaelnabil230 in https://github.com/laravel/framework/pull/50979
- [11.x] Allow time to be faked in database lock by @JurianArie in https://github.com/laravel/framework/pull/50981
- [11.x] Introduce method
Http::createPendingRequest()
by @Jacobs63 in https://github.com/laravel/framework/pull/50980 - [11.x] Add @throws to some doc blocks by @saMahmoudzadeh in https://github.com/laravel/framework/pull/50969
- [11.x] Fix PHP_MAXPATHLEN check for existing check of files for views by @joshuaruesweg in https://github.com/laravel/framework/pull/50962
- [11.x] Allow to remove scopes from BelongsToMany relation by @plumthedev in https://github.com/laravel/framework/pull/50953
- [11.x] Throw exception if named rate limiter and model property do not exist by @mateusjatenee in https://github.com/laravel/framework/pull/50908