Model casts in Laravel 10 are defined via the $casts
array property. However, in Laravel 11, you can define a casts()
method, which opens the possibility to use static methods on built-in casters as well as define static methods for custom casters:
use App\Enums\UserOption;use Illuminate\Database\Eloquent\Casts\AsEnumCollection; // ... /** * Get the attributes that should be cast. * * @return array<string, string> */protected function casts(): array{ return [ 'email_verified_at' => 'datetime', 'password' => 'hashed', 'options' => AsEnumCollection::of(UserOption::class), ];}
In Laravel 10, the same cast would look like the following since you cannot call static methods when defining an array property:
protected $casts = [ 'options' => AsEnumCollection::class.':'.UserOption::class,];
This update is backward-compatible with Laravel 10, and you can still define casts via the $casts
property combined with the new casts()
method. The $casts
property and casts()
method are merged, with the method keys taking precedence over the $casts
property.
I would recommend moving casts over to the method version if you want to take advantage of the static methods for the built-in casters.
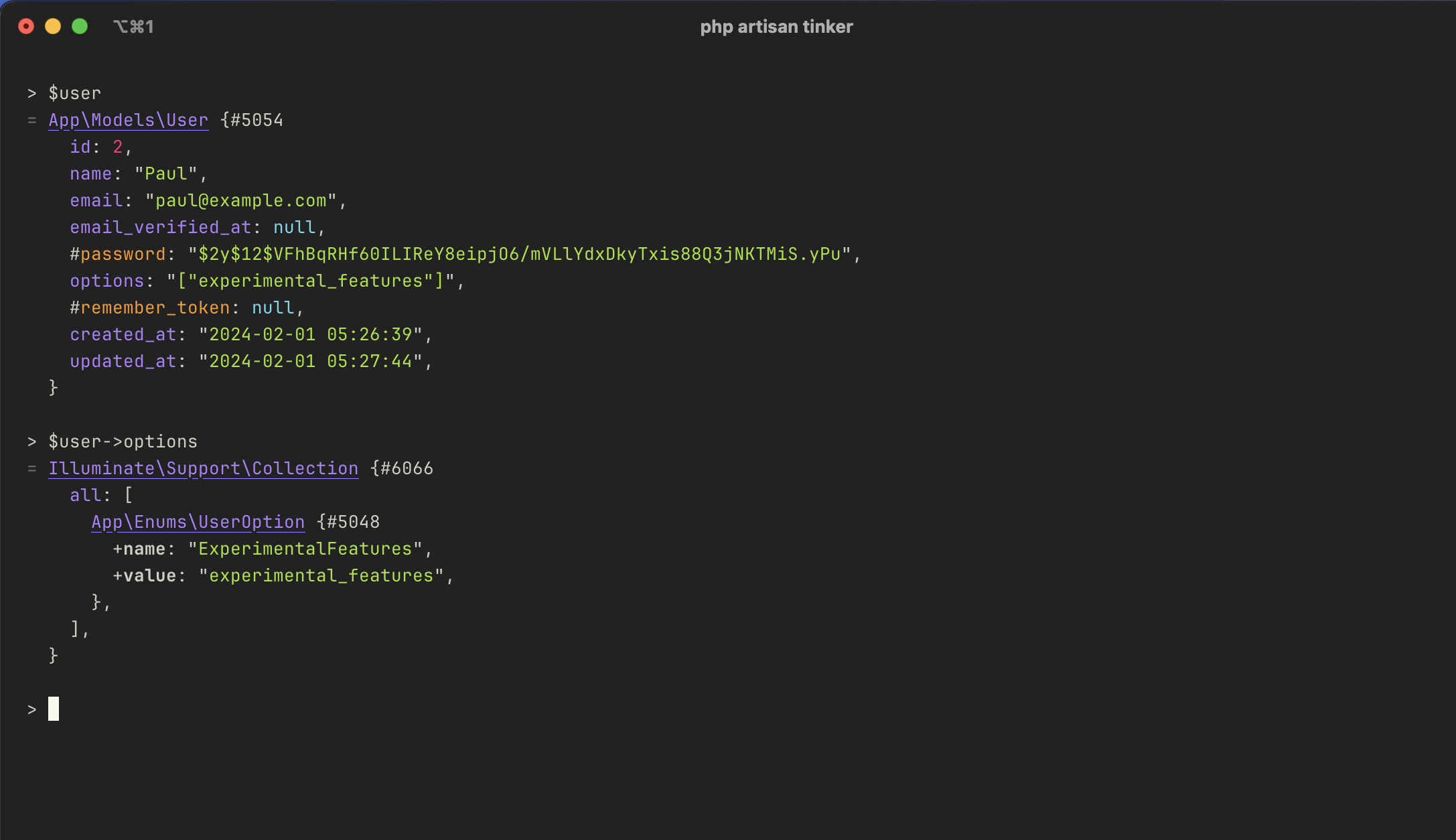
AsEnumCollection
casterAlong with the update to define casts via the casts()
method, new static methods were added to the built-in casters to ease defining casts:
AsCollection::using(OptionCollection::class);AsEncryptedCollection::using(OptionCollection::class);AsEnumArrayObject::using(OptionEnum::class);AsEnumCollection::using(OptionEnum::class);
Shout out to Nuno Maduro, who implemented this feature in Pull Request #47237!