PHP 8.3 is released with typed class constants, a json_validate function, and more
Published on by Paul Redmond
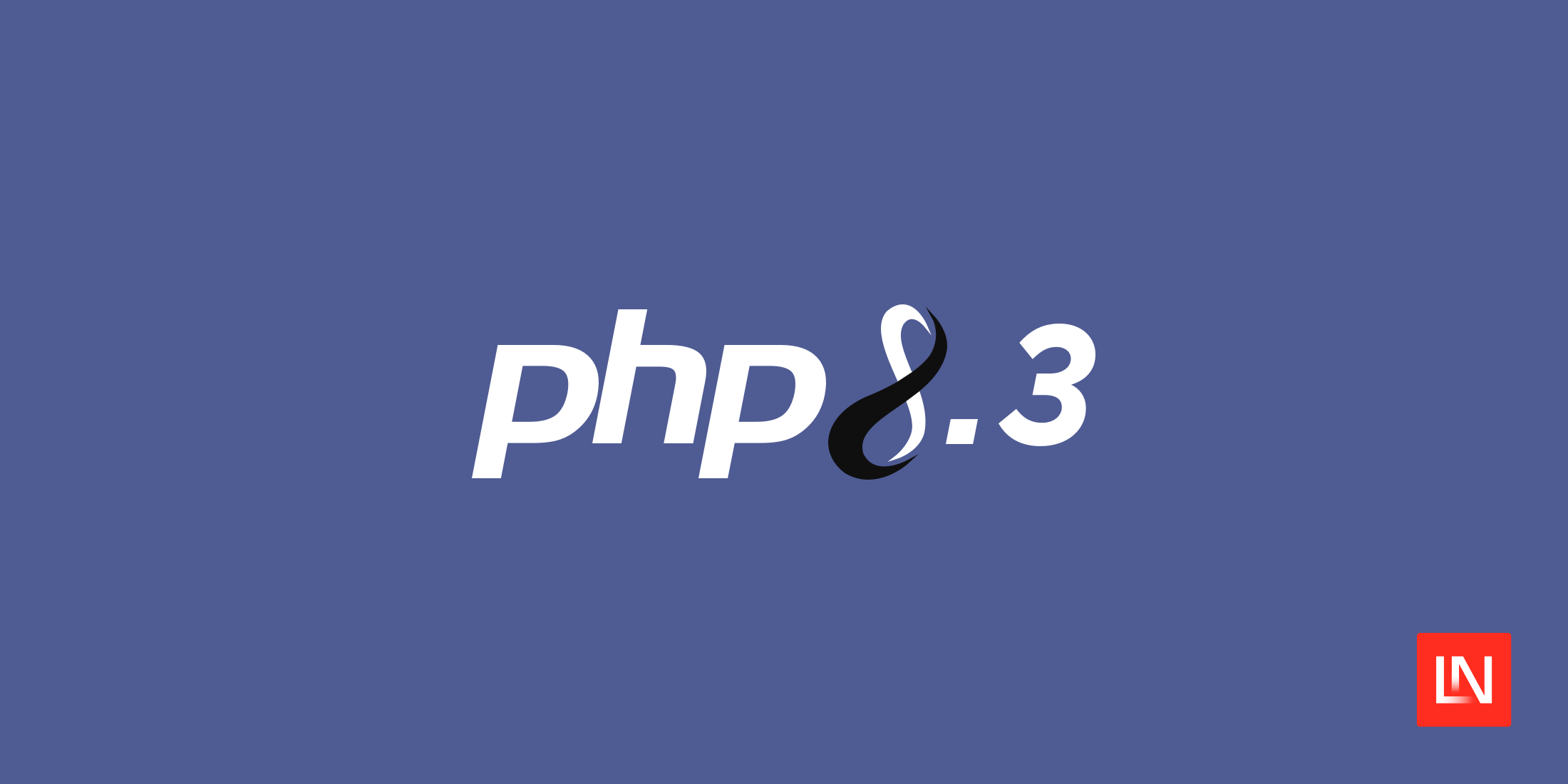
The PHP team has released PHP 8.3 today with typed class constants, a json_validate() function, dynamically fetching a class constant, the #[Override]
attribute, and more:
- Typed Class Constants
- json_validate() function
- Dynamic class constant fetch
- Marking overridden methods
- mb_str_pad() function
- Fallback value syntax for INI ENV variables
- Support linting multiple files at once
- More Appropriate Date/Time Exceptions
- Deep-cloning of readonly properties
- Randomizer Additions
- and more...
Typed Class Constants
In PHP 8.2, it was still not possible to declare constant (const
) types, which can lead to confusion or implications about the type you're working with:
interface I { const TEST = "Test"; // We may naively assume that the TEST constant is always a string} class Foo implements I { const TEST = []; // But it may be an array...} class Bar extends Foo { const TEST = null; // Or null}
Here's an example of how typed constants look in PHP 8.3:
interface I { const string TEST = E::TEST; // I::TEST is a string as well} class Foo implements I { use T; const string TEST = E::TEST; // Foo::TEST must also be a string} class Bar extends Foo { const string TEST = "Test2"; // Bar::TEST must also be a string, but the value can change} // Error example // Fatal error: Cannot use array as value for class constant// Foo::PHP of type stringclass Buzz implements I { const string PHP = [];}
The json_validate() function
To validate JSON in PHP, you would need to either configure the JSON_THROW_ON_ERROR
flag, use json_last_error
, or even just check for null
on a json_decode()
call:
json_decode(json: '{"foo": "bar}', flags: JSON_THROW_ON_ERROR); // JsonException Control character error, possibly incorrectly encoded.
As of PHP 8.3, you can also validate JSON using the json_validate()
function:
// Validjson_validate('{"framework": "Laravel"}'); // true // Invalidjson_validate('{"framework": "Laravel}'); // false json_last_error_msg(); // Control character error, possibly incorrectly encodedjson_last_error(); // 3
Dynamic class constant fetch
In PHP >= 8.2, dynamically fetching a class constant value was only possible using the constant()
function. The following would result in a syntax error:
class Framework { const NAME = 'Laravel';} $name = 'NAME'; // You could achieve this with the constant() functionconstant(Framework::class . '::' . $name); // Laravel // This following is a syntax error in >=v8.2.0echo Framework::{$name};// ParseError syntax error, unexpected token ";", expecting "(".
As of PHP 8.3, you can now access constants dynamically from a class,
class Framework { const NAME = 'Laravel';} $name = 'NAME'; // Syntax error in <= v8.2.0echo Framework::{$name}; // Laravel
Fallback value for environment variables in INI files
One of my favorite additions to PHP 8.3 is providing a default value when using environment variables to define INI settings. This will simplify Docker defaults without having to specify the defaults as ENV
blocks in a Dockerfile
. I am sure there are plenty of use cases out there that will be simplified by this.
Let's say, for example, that you want to allow a DRUPAL_FPM_PORT
ENV value to configure an INI value for the www
FPM pool:
error_log = syslogdaemonize = false [www]listen = localhost:${DRUPAL_FPM_PORT}
The DRUPAL_FPM_PORT
must be defined, and no default was possible! Now, you can do the following, which should feel familiar to a bash/shell script:
[www]listen = localhost:${DRUPAL_FPM_PORT:-9000}
Imagine shipping an xdebug.ini
file as part of your Dockerfile for development with sensible defaults yet allowing developers to override values they see fit.
Randomizer Additions
PHP 8.3 includes Randomizer Additions, including a new getBytesFromString()
method, which is convenient to get random bytes from a source string:
$randomizer = new \Random\Randomizer(); printf( "%s.example.com", $randomizer->getBytesFromString('abcdefghijklmnopqrstuvwxyz0123456789', 16)); // 3zsw04eiubcf82jd.example.com // Generate a random code for multi-factor authentication$randomizer = new \Random\Randomizer(new \Random\Engine\Secure()); echo implode('-', str_split($randomizer->getBytesFromString('0123456789', 20), 5)); // 11551-80418-27047-42075
Learn more
To get up to speed on these new features, check out the PHP 8.3.0 Release Announcement page for examples before/after PHP 8.3. Be sure to check out the deprecations and backward compatibility breaks.